1c find the second occurrence in the string. New String Functions
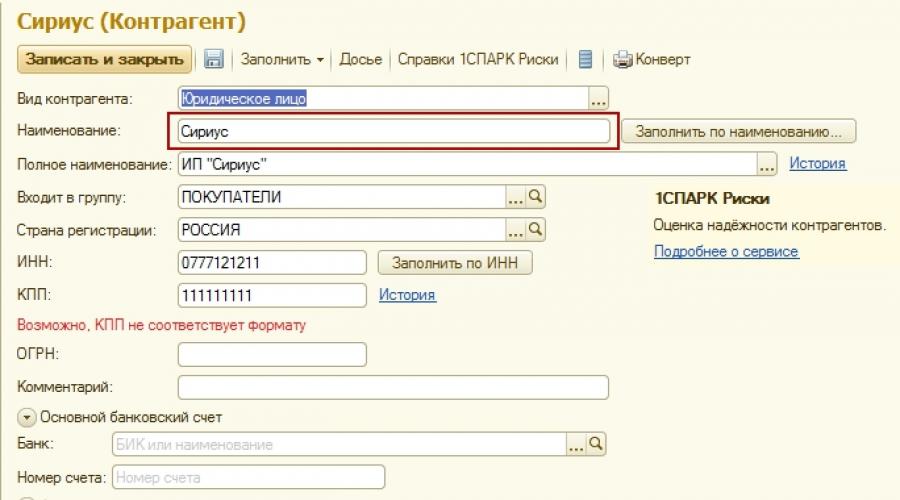
Read also
Special characters in 1C 8.3 - set special characters for string data types.
Let's consider each of them in more detail.
Special characters in 1C: VK, VTab, NPP, PS, PF, Tab
Special characters are called using the global property "Characters", for example:
Text = "Text before tab" + Symbols.Tab + "text after tab";
Get 267 1C video lessons for free:
- VC (CR) - carriage return. In the old days on dot-matrix or daisy-wheel printers, this character code was used as a command that returned the print head to the beginning of the line.
- NSP (NBSp) - non-breaking space (often seen in numeric strings, for example: 1000).
- PS (LF) - Line feed (LF - linefeed) - scroll the drum one line down, the print head is in the same place.
- PF (FF) - format translation. The sheet is ejected from the printer, printing starts from a new sheet.
- Tab (Tab) - a tab character. Horizontal tabs - a way to position for printing on the nearest column, a multiple of some number (for example, 8, 16, 24 ...).
- VTab (VTab) - vertical tabulation. It is similar to horizontal, only we are positioned on the line. Causes some scrolling of the printer drum (printhead stays in the same position (column)!)
PS + VK is a combination that performs two operations: scrolls the drum down a line and returns the print carriage to the beginning of the line, which corresponds to what the user expects to receive by pressing Enter.
If you are starting to learn 1C programming, we recommend our free course(do not forget
String is one of the primitive data types in 1C:Enterprise 8 systems. Variables with type line contain text.
Type Variable Values line are enclosed in double quotes. Multiple Variables of this type can be folded.
Per1 = "Word 1" ;
Per2 = "Word 2" ;
Per3 = Per1 + " " + Per2 ;
Eventually Per3 will matter " Word 1 Word 2″.
In addition, 1C:Enterprise 8 systems provide functions for working with strings. Consider the main ones:
EnterString(<Строка>, <Подсказка>, <Длина>, <Многострочность>) — the function is designed to display a dialog box in which the user can specify the value of a variable of type Line. Parameter <Строка> is required and contains the name of the variable into which the entered string will be written. Parameter <Подсказка> optional is the title of the dialog box. Parameter <Длина> optional, indicates the maximum length of the input string. The default is zero, which means unlimited length. Parameter <Многострочность> optional. Specifies the multiline text input mode: True — multiline text input with line separators; False - Enter a simple string.
A string can be entered and, knowing the character code in Unicode:
Symbol(<КодСимвола>) — The code is entered as a number.
Letter= Symbol(1103 ) ; // I
There is also an inverse function that allows you to find out the code of a character.
SymbolCode(<Строка>, <НомерСимвола>) — Returns the Unicode number of the specified character as a number.
Text case conversion functions:
VReg(<Строка>) - Converts all characters in a string to uppercase.
HReg(<Строка>) - Converts all characters in a string to lower case.
TReg(<Строка>) - converts all characters of the string to title case. That is, the first letters in all words are converted to uppercase, and the remaining letters are converted to lowercase.
Functions for searching and replacing characters in a string:
Find(<Строка>, <ПодстрокаПоиска>) - finds the character number of the occurrence of the search substring. For example:
Find ("String" , "eye" ) ; // 4
StrFind(<Строка>, <ПодстрокаПоиска>, <НаправлениеПоиска>, <НачальнаяПозиция>, <НомерВхождения>) — finds the character number of the occurrence of the search substring, the occurrence number is specified in the corresponding parameter. In this case, the search starts from the character, the number of which is specified in the parameter StartPosition. The search is possible from the beginning or from the end of the string. For example:
Number4 Entry= StrFind( "Defensiveness", "o" , Search Direction. First, 1 , 4 ) ; // 7
StrReplace(<Строка>, <ПодстрокаПоиска>, <ПодстрокаЗамены>) Finds all occurrences of the search substring in the source string and replaces it with the replacement substring.
StrReplace("String" , "eye" , "" ) ; // Page
Empty line(<Строка>) - checks the string for significant characters. If there are no significant characters, or no characters at all, then the value is returned True. Otherwise - Lie.
StrNumberOccurrences(<Строка>, <ПодстрокаПоиска>) – calculates the number of occurrences of the search substring in the source string.
StrNumberOccurrences ( "Study, study and study again", "study" , "" ) ; // 3
StrTemplate(<Строка>, <ЗначениеПодстановки1>…<ЗначениеПодстановкиN> — substitutes the parameters into the string by number. The string must contain substitution markers of the form: "%1..%N". Numbering of markers starts from 1. If the parameter value Undefined, the empty string is substituted.
StrPattern ( "Option 1 = %1, Option 2 = %2", "1" , "2" ) ; // Parameter 1= 1, Parameter 2 = 2
String conversion functions:
A lion(<Строка>, <ЧислоСимволов>) returns the first characters of the string.
Right(<Строка>, <ЧислоСимволов>) - returns the last characters of the string.
Wednesday(<Строка>, <НачальныйНомер>, <ЧислоСимволов>) - returns a string of length<ЧислоСимволов>, starting with a character<НачальныйНомер>.
Abbr(<Строка>) —truncates non-significant characters to the left of the first significant character in the string.
abbr(<Строка>) - cuts off insignificant characters to the right of the last significant character in the string.
Abbrl(<Строка>) - cuts off non-significant characters to the left of the first significant character in the string and to the right of the last significant character in the string.
StrGetString(<Строка>, <НомерСтроки>) – gets the string of a multiline string by number.
Other features:
StrLength(<Строка>) - returns the number of characters in a string.
StrNumber of Lines(<Строка>) - returns the number of rows in a multiline string. A line is considered new if it is separated from the previous line by a newline character.
StrCompare(<Строка1>, <Строка2> ) - compares two strings case insensitively. The function works like an object Comparing Values. Returns:
- 1 - if the first line is greater than the second
- -1 - if the second line is greater than the first
- 0 - if strings are equal
StrCompare("First row" , "Second row" ) ; // 1
The String type is found in all programming languages. It is primitive, and in 1C there are many functions for working with it. In this article, we will take a closer look at various ways work with string types in 1C 8.3 and 8.2 with examples.
Line
In order to convert a variable of any type to a string, there is the "String ()" function of the same name. The input parameter will be the variable itself, the string representation of which is to be obtained.
String(False) // returns "No"
String(12345) // returns "12 345"
String(CurrentDate()) //"21.07.2017 11:55:36"
It is possible to convert to a string not only primitive types, but also others, for example, elements of directories and documents.
Abbreviated LP, Abbreviated L, Abbreviated P
The input parameters of these functions are a string type variable. Functions remove insignificant characters (spaces, carriage returns, etc.): from the left and right side, only on the left side, and only on the right, respectively.
abbrl("Spaces on both sides will be removed") // "Spaces on both sides will be removed"
abbr("Spaces on both sides will be removed") // "Spaces on the left will be removed"
abbr(" Spaces on both sides will be removed ") // "Spaces on the right will be removed"
Leo, Right, Middle
These functions allow you to cut off part of a string. The Lion() function will return the part of the string on its left side of the specified length. The "Right()" function is similar, but cropping is done on the right. The "Wed()" function allows you to specify the character number from which the string will be selected and its length.
Lion("String variable", 4) // returns "Stro"
Right("String variable", 7) // returns "variable"
avg("String variable", 2, 5)// returns "troco"
StrLength
The function determines the number of characters that are contained in a string variable.
StrLength("Word") // the result of execution will be the number 5
Find
The function makes it possible to search for a part of a string in a string variable. The return value will be a number that indicates the position of the beginning of the found string. If no matches are found, zero is returned.
Note that the search is case sensitive. If there is more than one occurrence of the search substring in the original string, the function will return the beginning of the first occurrence.
Find("one, two, one, two, three", "two") // the function will return the number 6
Empty line
Using this function allows you to determine if a string is empty. Insignificant characters, such as space, carriage return, and others are not taken into account.
EmptyString("Vasily Ivanovich Pupkin") // the function will return False
EmptyString(" ") // the function will return True
VReg, NReg, TReg
These functions are very useful when comparing and converting string variables. "Vreg()" will return the original string in uppercase, "HReg()" in lowercase, and "TReg()" will format it so that the first character of each individual word is capitalized, and all subsequent characters are lowercase.
VReg("GENERAL DIRECTOR") // return value - "GENERAL DIRECTOR"
HReg("GENERAL DIRECTOR") // return value - "CEO"
TReg("GENERAL DIRECTOR") // return value - "General Director"
StrReplace
This function is an analogue of the replacement in text editors. It allows you to substitute one character or set of characters for another in string variables.
StrReplace("red, white, yellow", ",", ";") // returns "red; white; yellow"
StrNumberRows
The function allows you to determine the number of lines separated by a carriage return in a text variable.
The loop in the example below will go through three circles because the StrNumberRows function will return the value 3:
For ind \u003d 1 by StrNumber of Lines ("Line1" + Symbols.PS + "String2" + Symbols.PS + "Line3") Loop
<тело цикла>
EndCycle;
StrGetString
This function works with multiline text in the same way as the previous one. It allows you to get a specific string from a text variable.
StrGetString("String1" + Symbols.PS + "String2" + Symbols.PS + "String3", 2) // returns "Line2"
StrNumberOccurrences
The function counts the number of occurrences of a character or substring in the searched string.
StrNumberInstallations("a;b;c;d; ", ";") // the function will return the number 4
Symbol and SymbolCode
These functions allow you to get a character by its Unicode code, as well as determine this code by the character itself.
SymbolCode("A") // the function will return the number 1 040
SymbolCode(1040) // the function will return "A"
Frequent tasks when working with strings
String Concatenation
To concatenate multiple strings (concatenate) just use the addition operator.
"Line 1" + "Line 2" //the result of adding two lines will be "Line 1 Line 2"
Type conversion
In order to convert a type to a string, for example, a reference to a dictionary element, a number, and so on, it is enough to use the "String ()" function. Functions like "ShortLP()" will also convert variables to a string, but immediately with cutting off insignificant characters.
String(1000) // will return "1000"
Please note that when converting a number to a string, the program automatically added a space separating the thousand. In order to avoid this, you can use the following structures:
StrReplace(String(1000),Characters.NPP,"") // returns "1000"
String(Format(1000,"CH=")) // will return "1000"
Quotes in a string
Quite often you will have to deal with the need to put quotes in a string variable. It can be either a request text written in the configurator, or just a variable. To solve this problem, you just need to set two quote characters.
Header = String("Horns and Hooves LLC is us!") // returns "Roga and Hooves LLC is us!"
Multiline, line break
In order to create multiline text just add line break characters to it (Symbols.PS).
MultilineText = "First Line" + Characters.PS + "Second Line"
How to remove spaces
In order to remove spaces on the right or left, you can use the function "Stretch()" (as well as "Scrpt()" and "ScreenP()"):
StringWithoutSpaces = ShortLP(" Many letters ") // the function will return the value "Many letters"
If, after converting a number to a string, you need to remove non-breaking spaces, use the following construct:
StringWithoutSpaces = StrReplace(String(99999),Characters.NPP,"") // returns "99999"
Also, programmers often use the following construction, which allows you to remove or replace all spaces of a text variable with another character:
StringWithoutSpaces = StrReplace(" hello", " " ,"") // returns "hello"
Comparing strings to each other
You can compare terms with the usual equal sign. The comparison is case sensitive.
"hello" = "hello" // will return false
"Hello" = "Hello" // will return True
"Hello" = "Goodbye" // will return False
Strings in 1C 8.3 in the built-in language 1c are values of a primitive type Line. Values of this type contain a Unicode string of arbitrary length. String type variables are a set of characters enclosed in quotation marks.
Example 1. Let's create a string variable with text.
StringVariable = "Hello world!";
Functions for working with strings in 1s 8.3
This section will provide the main functions that allow you to change lines in 1s, or analyze the information contained in them.
StrLength
StrLength(<Строка>) . Returns the number of characters contained in the string passed in the parameter.
Example 2. Let's count the number of characters in the string "Hello world!".
String = "Hello world!"; Number of Characters = StrLength(String); Report(Number of Characters);
The result of executing this code will be displaying the number of characters in the string: 11.
Abbreviated
Abbr(<Строка>)
. Trims non-significant characters to the left of the first significant character in a string.
Insignificant characters:
- space;
- non-breaking space;
- tabulation;
- carriage return;
- line translation;
- translation of the form (page).
Example 3. Remove all spaces from the left side of the string "peace!" and append the string "Hello" to it.
String = abbr("world!"); String = "Hi"+String; Notify(String);
The result of the execution of this code will be the display of the string "Hello world!".
Abbreviation
abbr(<Строка>) . Trims non-significant characters to the right of the first significant character in a string.
Example 4. Form from the strings "Hello" and "world!" phrase "Hello world!"
String = abbr("Hi")+" "+abbr("world!"); Notify(String);
Abbreviated LP
Abbrl(<Строка>)
. Trims non-significant characters to the right of the first significant character in the string, also trims non-significant characters to the left of the first significant character in the string. This function is used more often than the previous two, as it is more versatile.
Example 5. Remove insignificant characters on the left and right in the name of the counterparty.
Contractor = Directories.Contractors.FindBy Details("TIN", "0777121211"); ContractorObject = Contractor.GetObject(); ContractorObject.Description = Abbreviated LP(ContractorObject.Description); ContractorObject.Write();
a lion
A lion(<Строка>, <ЧислоСимволов>)
. Gets the first characters of a string, the number of characters is specified in the parameter Number of Characters.
Example 6. Let the structure Employee contain the name, surname and patronymic of the employee. Get string with last name and initials.
InitialName = Lion(Employee.Name, 1); Patronymic Initial = Leo(Employee.Patronymic, 1); FullName = Employee.LastName + " " + FirstName Initial + "." + Patronymic Initial + ".";
Rights
Right(<Строка>, <ЧислоСимволов>) . Gets the last characters of a string, the number of characters is specified in the parameter Number of Characters. If the specified number of characters exceeds the length of the string, then the entire string is returned.
Example 7. Let a date be written at the end of a string variable in the format “yyyymmdd”, get a string with a date and convert it to type date.
String = " The current date: 20170910"; StringDate = Rights(String, 8); Date = Date(StringDate);
Wednesday
Wednesday(<Строка>, <НачальныйНомер>, <ЧислоСимволов>) . Gets a substring from the string passed in the parameter Line, starting from the character whose number is specified in the parameter InitialNumber and the length passed to the parameter Number of Characters. The numbering of characters in a string starts from 1. If the parameter InitialNumber a value less than or equal to zero is specified, then the parameter takes the value 1. If the parameter Number of Characters is not specified, characters up to the end of the string are selected.
Example 8. Let the string variable contain the region code starting from the ninth position, you should get it and write it in a separate line.
String = "Region: 99 Moscow"; Region = Avg(Row, 9, 2);
PageFind
StrFind(<Строка>, <ПодстрокаПоиска>, <НаправлениеПоиска>, <НачальнаяПозиция>, <НомерВхождения>) . Searches for the specified substring in a string, returns the position number of the first character of the found substring. Consider the parameters of this function:
- Line. Source string;
- SubstringSearch. The desired substring;
- DirectionSearch. Specifies the direction to search for a substring in a string. Can take values:
- Search Direction.From the Beginning;
- Search Direction.From the End;
- StartPosition. Specifies the position in the string at which to start the search;
- Entry Number. Specifies the number of occurrences of the searched substring in the source string.
Example 9. In the line "Hello world!" determine the position of the last occurrence of the "and" character.
PositionNumber = StrFind("Hello World!", "and", SearchDirection.From End); Notify(PositionNumber);
The result of the execution of this code will be the display of the number of the last occurrence of the symbol "and" on the screen: 9.
VReg
VReg(<Строка>) . Converts all characters of the specified string to 1s 8 to upper case.
Example 10. Convert the string "hello world!" to uppercase.
StringVreg = Vreg("hello world!"); Report(StringVreg);
The result of the execution of this code will be the display of the string "HELLO WORLD!"
HReg
HReg(<Строка>) . Converts all characters of the specified string to 1s 8 to lower case.
Example 11. Convert the string "HELLO WORLD!" to lower case.
StringNreg = NReg("HELLO WORLD!"); Report(StringVreg);
The result of the execution of this code will be the display of the string "hello world!"
TReg
TReg(<Строка>) . Converts a string as follows: the first character of each word is converted to uppercase, the remaining characters of the word are converted to lowercase.
Example 12. Capitalize the first letters of the words in the string "hello world!".
StringTreg = TReg("hello world!"); Report(StringTreg);
The result of the execution of this code will be the display of the string "Hello World!"
Symbol
Symbol(<КодСимвола>) . Gets a character by its Unicode code.
Example 13. Add left and right to the line "Hello World!" symbol ★
StringWithStars = Character("9733")+"Hello World!"+Character("9733"); Report(StringWithStars);
The result of the execution of this code will be the display of the string "★Hello World!★"
SymbolCode
SymbolCode(<Строка>, <НомерСимвола>) . Gets the Unicode character code from the string specified in the first parameter, located at the position specified in the second parameter.
Example 14. Find out the code of the last character in the string "Hello World!".
String = "Hello World!"; CharacterCode =CharacterCode(String, StrLength(String)); Notify(CharacterCode);
The result of the execution of this code will be the display of the code of the symbol "!" — 33.
Empty line
Empty line(<Строка>) . Checks if the string consists of only non-significant characters, that is, if it is empty.
Example 15. Check if the string is empty and consists of three spaces.
Empty = EmptyString(" "); Report(Empty);
The result of the execution of this code will be the display of the word "Yes" (string expression of a boolean value True).
StrReplace
StrReplace(<Строка>, <ПодстрокаПоиска>, <ПодстрокаЗамены>) . Finds all occurrences of the search substring in the source string and replaces it with the replacement substring.
Example 16. In the line "Hello World!" replace the word "World" with the word "Friends".
String = StrReplace("Hello World!", "World", "Friends"); Notify(String);
The result of the execution of this code will be the display of the string "Hello Friends!"
StrNumberRows
StrNumber of Lines(<Строка>) . Allows you to count the number of lines in a multiline string. To move to a new line in 1s 8, the symbol is used PS(line feed character).
Example 17. Determine the number of lines in the text:
"First line
Second line
Third line"
Number = StrNumber of Lines("First line"+Symbols.PS +"Second line"+Symbols.PS +"Third line"); Report(Number);
The result of the execution of this code will be the display of the number of lines in the text: 3
StrGetString
StrGetString(<Строка>, <НомерСтроки>) . Gets a string in a multiline string by its number. Line numbering starts from 1.
Example 18. Get the last line in the text:
"First line
Second line
Third line"
Text = "First Line"+Characters.PS +"Second Line"+Characters.PS +"Third Line"; LastLine = StrGetLine(Text, StrNumber of Lines(Text)); Notify(Last Line);
The result of the execution of this code will be the display of the line "Third line".
StrNumberOccurrences
StrNumberOccurrences(<Строка>, <ПодстрокаПоиска>) . Returns the number of occurrences of the specified substring in a string. The function is case sensitive.
Example 19. Determine how many times the letter “c” enters the line “Lines in 1s 8.3 and 8.2”, regardless of its case.
String = "Lines in 1s 8.3 and 8.2"; Number of Occurrences = StrNumber of Occurrences(Vreg(String), "C"); report(number of occurrences);
The result of executing this code will display the number of occurrences on the screen: 2.
PageBeginsFrom
StrBeginsFrom(<Строка>, <СтрокаПоиска>) . Checks if the string given in the first parameter begins with the string in the second parameter.
Example 20. Determine if the TIN of the selected counterparty begins with the number 1. Let the variable counterparty Counterparties.
TIN = Contractor.TIN; StartsCUnits = StrStartsC(TIN, "1"); If StartsFROM1 Then //Your code EndIf;
Page Ends On
StrEndsOn(<Строка>, <СтрокаПоиска>) . Checks if the string passed in the first parameter ends with the string in the second parameter.
Example 21. Determine if the TIN of the selected counterparty ends with the number 2. Let the variable counterparty the link to the directory element is stored Counterparties.
TIN = Contractor.TIN; Ends With Two = Str Ends With (TIN, "2"); If Ends With Two Then //Your Code EndIf;
PageSplit
StrDivide(<Строка>, <Разделитель>, <ВключатьПустые>) . Splits a string into parts by the specified delimiter characters and writes the resulting strings to an array. The first parameter stores original string, in the second a string containing a separator, in the third it is indicated whether it is necessary to write to an array empty lines(default True).
Example 22. Suppose we have a string containing numbers separated by the symbol ";", get an array of numbers from the string.
String = "1; 2; 3"; Array = StrSplit(String, ";"); For Count = 0 By Array.Quantity() - 1 Loop Attempt Array[Count] = Number(Ablp(Array[Count])); Exception Array[W] = 0; EndTry EndCycle;
As a result of execution, an array with numbers from 1 to 3 will be obtained.
StrConnect
StrConnect(<Строки>, <Разделитель>) . Converts an array of strings from the first parameter to a string containing all the elements of the array using the delimiter specified in the second parameter.
Example 23. Using the array of numbers from the previous example, get the original string.
For Count = 0 By Array.Quantity() - 1 Loop Array[Count] = String(Array[Count]); EndCycle; String = StrConnect(Array, "; ");
Implemented in version 8.3.6.1977.
We have expanded the set of functions for working with strings. We did this in order to give you more advanced tools for parsing string data. New functions will be convenient and useful in technological tasks of text analysis. In tasks related to the parsing of text that contains data in a formatted form. This may be the analysis of some files received from the equipment, or, for example, the analysis of a technological log.
All the actions that the new functions perform, you could perform before. With the help of more or less complex algorithms written in an embedded language. Therefore, new functions do not give you any fundamentally new opportunities. However, they allow you to reduce the amount of code, make the code simpler and more understandable. In addition, they allow you to speed up the execution of actions. Because the functions implemented in the platform work, of course, faster than a similar algorithm written in the built-in language.
Format function StrTemplate()
This function substitutes parameters into a string. The need for such a conversion often arises, for example, when displaying warning messages. The syntax for this function is as follows:
StrTemplate(<Шаблон>, <Значение1-Значение10>)
<Шаблон>is the string in which to substitute the parameter representations.
<Значение1> , ... <Значение10>- these are the parameters (maximum - ten), the representations of which must be substituted into the string.
To specify a specific place in the template to which you want to perform the substitution, you need to use markers of the form %1, ... %10. The number of markers involved in the template and the number of parameters containing values must match.
For example, the result of executing such an operator:
there will be a line:
Data error on line 2 (requires Date type)
String Function StrCompare()
This function compares two strings case insensitively. For example, like this:
You could perform the same action before using the ValueComparison object:
However, using the new function looks simpler. And besides, the function, unlike the Value Compare object, works both in the thin client and in the web client.
String Functions StrBeginsC(), StrEndsTo()
These functions determine whether a string starts with a specified substring, or whether a string ends with a specified substring. The algorithm of these functions is not difficult to implement in a built-in language, but their presence allows you to write cleaner and more understandable code. And they work faster.
For example, they are convenient to use in the If statement:
Functions for working with strings StrSplit(), StrJoin()
These functions split the string into parts according to the specified delimiter. Or vice versa, they combine several lines into one, inserting the selected separator between them. They are convenient for creating or analyzing logs, a technological log. For example, you can easily disassemble a technological log entry into parts suitable for further analysis:
Function of working with strings StrFind()
Instead of the old Find() function, we implemented new feature, which has additional features:
- Search in different directions (from the beginning, from the end);
- Search from the specified position;
- Search for an occurrence with specified number(second, third, etc.).
In fact, it duplicates the capabilities of the old function. This is done in order to maintain compatibility with modules compiled in older versions. The old Find() function is recommended not to be used anymore.
Below is an example using the new search capabilities. Searching backwards is useful when you need the last fragment of a formalized string, for example, full name file in URL. And searching from a specified position helps in cases where you need to search in a known fragment, and not in the entire string.