What does read mean in pascal. Data input statements ReadLn and Read
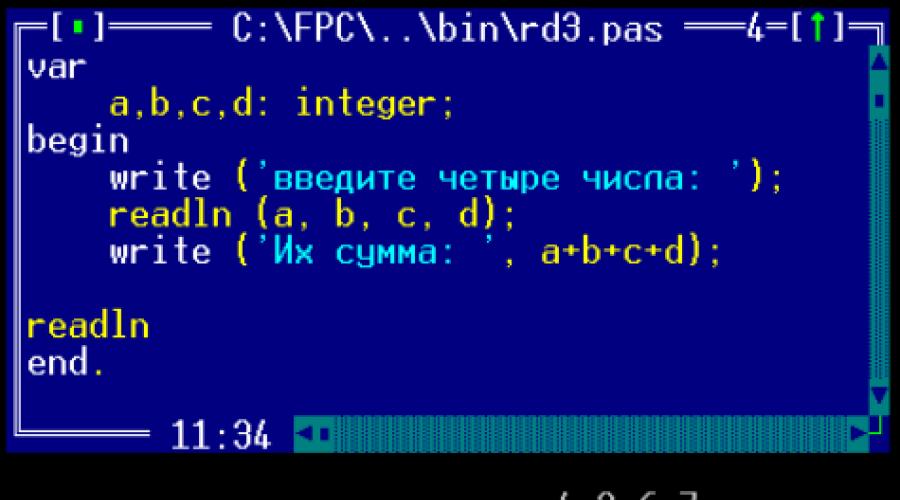
Read also
You are in the section of materials on programming in Pascal. Before we start programming, we need to clarify some concepts that we will need at the beginning. You can't just program like that. We cannot write the program in words - the computer, apart from zeros and ones, does not understand anything else. To do this, Pascal created a special symbolism - the Pascal language, a set of reserved words that cannot be used in their programs anywhere else, except for their intended purpose. We list the basic concepts that we need at the beginning:
✎ 1) program - in English “program”, written at the very beginning of the code, followed by the name of the program in Latin and a semicolon. For example: program Summa; − a program called Summa. But this part of the code, called the program header, can not be written - it is present only for clarity and shows what problem it solves this program. Here we used the word "code" - this is the name text entry programs.
✎ 2) integer - in English it means "integer" (or simply "integer") and in Pascal it is used to denote 32-bit (8 bytes) signed integers from the range [-2147483648, 2147483647] . What these large numbers mean, we will analyze later.✎ 3) real - from English “real”, “real”, “real”, “real”. In Pascal, this term refers to real numbers from the range [-1.8∙10 308 , 1.8∙10 308 ] . These are very large numbers, but 15 - 16 significant digits are displayed. By the way, integer and real data types in the environment Pascal programming ABC.Net is always automatically highlighted in blue.
✎ 4) const - analogue of English. "constant", meaning "constant", "constant". In Pascal, this is a value that cannot be changed. It is written like this:
This entry should be taken as it is written: the number N is equal to 12, S is equal to 5, "pi" is equal to 3.14 (as in mathematics, only a dot is used instead of a comma in Pascal). In the last line, we used a double slash (two slashes), followed by text - this is how comments are written in Pascal, and the program does not perceive them. Everything starting with a double slash and up to the end of the line is a comment that is written to explain the program and is always highlighted in a different color (in PascalABC.Net it is green, in Turbo Pascal this type of comment is not used). There is another type of comment - this (text enclosed in curly brackets, just like here, also highlighted in green) . This type of comment can act for several lines in a row - from the beginning of the bracket to its closing, and the compiler does not perceive everything that is in the middle of such a construction as code and simply skips it.
In fact, the recording format const a little more difficult. According to the rules, we had to write:
|
After declaring each value, its type is specified, and then a value is assigned. But the previous entry is also correct, because the Pascal compiler is configured to automatically determine the type of the constant. But this cannot be said about the next type of numbers - variables.
✎ 5) var - comes from English. “variable” (“variable”, or “changeable”), which in Pascal means a value that can change its value during the program. It is written like this:
As can be seen from the entry, there is no “=” sign here - variables of the same type are recalculated (separated by commas) and only the type is indicated after the colon. Variables N, m (integer) and Q, r, t (real) in the program can change values within integer and real respectively. One more note: the description of variables always comes after the description of constants (constant) - first comes the construction const , and then var .
✎ 6) begin - translated from English means “begin” and Pascal means the beginning of the main program in which commands (operators) are written. After the word begin semicolon is not included.
✎ 7) end - in English. "end" and Pascal language means the same (end of program). After the last word end there is always a dot. We highlighted the word "last" because the use of the construction begin-end perhaps in one more case: these are the so-called operator brackets, which are used to combine several operations under one operator. But more about that later. So the main program would look like this:
|
Here, the statements in the body of the program are different commands to the compiler.
✎ 8) write - in English means "to write." This statement displays the text placed in it, which is why it is called the output statement. The text placed in it is highlighted in blue and written like this:
Write( "this text is displayed on the screen");
The message in parentheses and quotes will be shown in the console window (you can't just put it in parentheses without quotes). After executing this statement, we will see on the screen:
this text is displayed on the screen
In this form, the write operator is used when it is necessary to show a hint, explanation, comment, etc. And from if it is also necessary to display a numerical value, say, S = 50 square meters. m, then the following format is used:
Write(, S);
As a result, we get the result on the screen:
The area value is: S = 50
And if you need to display units of measurement, you need to insert the text in quotation marks after S again:
Write( "The size of the area is: S = ", S, "sq.m" );
After executing the last output statement, we get the output on the screen:
The size of the area is: S = 50 sq.m
✎ 9) writeln - the same as write, but after execution, the cursor will move to the next line.
✎ 10) read - translated from English means "read", so read is called a readout operator, or data input. Written as read(N), which means that the value N must be entered, where N is any number, or text, or other type of variable. For example, if we need to enter the age of a person who is 32 years old, we can write it like this:
In the first line of this code, the program displays the question " What is your age?» and moves the cursor to the next line (ending ln); in the second line we print "Year =" (a space at the beginning); then we see the readln(Year) operator, which means the need to enter the Year age (number 32); finally, we display the messages “My age”, “32”, and “years. » in turn. You need to be careful about spaces. As a result of executing this code, we will receive a message:
What is your age?
Year = 32
My age is 32
✎ 11) readln - the same as read, only with a newline. Indeed, in the example above, after entering the number Year, we only write in the next line: “ My age is 32».
That's all for now. On the next page we will write our first program, and in Pascal programming these will be our
As well as for information output statements, the read and reeadln statements are operators for accessing built-in information entry procedures.
The operators read (read) and readln, which comes from two English words read (read) and line (line) are used in programs to enter information into computer memory and " readings" values into a variable.
Consider the work of these operators and procedures for entering information.
Our program has a readln(a) procedure. When executing a program, upon encountering a readln statement, the computer will pause while waiting for information to be entered. After we enter the value of the variable a - 16 from the keyboard, the computer will assign this value to the variable a, i.e. will send it to the memory location named a and continue the program execution. We call this process " reading" values into a variable.
So, the procedures read and readln "read" the values of variables and assign them to the variables that are written to them.
There can be several such variables, then they are written in these operators separated by commas, for example:
read(a, b, c, n, g, j, i), readln(e, f, k, p, d) etc.
What is the difference between read and readln procedures?
The read procedure will require after itself the input or output of information in one line, and the readln procedure makes it possible to enter and output information after itself from the beginning new line.
For example:
In the program: write("Enter the values a and b "); read(a,b);
write("Entering information in one line");
When this part of the program is executed, everything written in the first write statement will be displayed on the screen, then the cursor will be on the same line, and the computer will wait for the values a and b to be entered. Let's enter their values - 2 and 3, separating them with a space or, in other words, with a space. After that, the information written in the next write statement will be output on the same line.
On the screen:
Enter the values for a and b 2 3 Enter information on one line
In a programme:
writeln("Enter a, b, and c); readln(a, b, c);
writeln("Input and output information from the beginning of the line");
On the screen:
Enter the values a, b and c
Entering and outputting information from the beginning of a line
Arithmetic operations with integers. Integer type variables. Real type
In Pascal, integers are used, which include all natural numbers formed in the process of counting objects: 1, 2, 3, 4, 5, 6, ...; negative numbers: ..., -6, -5, -4, -3, -2, -1 and the number zero: 0. Integers form the following series:
6, -5, -4, -3, -2, -1, 0, 1, 2, 3, 4, 5, 6, ...
In Pascal, integers range from -32768 to 32767.
Variables, which take integer values, are written in the description section with an indication of the type integer (integer).
For example: var a, b, c, a1, b34, nomb: integer;
Values of a different type to these variables in the same program cannot be assigned.
Arithmetic operations with integers and variables of integer type in Pascal
The "_" sign means a space. Spaces between variable names and operation name (div) are required. (It comes from the English division - division).
The remainder of dividing a by b. a_mod_b
Subject: Input Output. Operators Read (Readln), Write (Writeln). The simplest linear programs.
We solve the problem by commenting on each of our actions in curly braces. Recall that the comment is not perceived by the computer, and we need it in order to better understand how the program works.
Task . Write a program that clears the screen and calculates the product of two numbers entered by the user.
ProgramProizv2; uses Crt;(Include Crt module) Var number1, (variable that will contain the first number) number2, (variable that will contain the second number) result (variable that will contain the result) : integer; Begin ClrScr;(Using screen clearing routine from Crt module) Write("Enter the first number"); readln(number1); (The number entered by the user is read into the variable number1) Write("Enter second number"); (We display characters written between apostrophes on the screen) readln(number2); (The number entered by the user is read into the variable number2) result:= number1 * number2; (We find the product of the entered numbers and assign the result to the variable) Write ("The product of the numbers ", number1, " and ", number2, "is equal to", rezult); (We display a line containing the answer to the problem) Readln;(Screen Delay Procedure) end. |
To better understand how a program works, type it on your computer and test it out. Answer the questions:
- why is the program called Proizv2?
- Why was the Crt module placed in the Uses section?
- what is the purpose of the variables number1, number2, result?
- what type are these variables? what does it mean?
- If we assign the values 5 and 7 to the variables number1 and number2, respectively, what line will the computer produce when the last Write procedure is executed? Write it down in your notebook.
- in which lines the user is asked for the values of variables?
- Which line is where the numbers are multiplied?
- what does the assignment operator do in this program?
Exercise . Modify the program so that it prompts the user for another variable and prints out the result of the product of three numbers.
Write and WriteLn statements
We have already used the Write and WriteLn operators, but we need to elaborate on the rules for using these operators.
Write (English write) - an operator that is used to display information on the screen. The WriteLn operator performs the same action, but since it also has the ending Ln (line - English line, line), after displaying the desired message on the screen, it additionally moves the cursor to the next line.
General form:
Write (list of expressions)
WriteLn (expression list)
The Write and WriteLn procedures are used not only to display the result, but also to display various messages or queries. This allows you to have a dialogue with the user, tell him when he needs to enter values, when he gets the result, when he made a mistake, etc.
For example, when executing the procedure WriteLn ('Found number', a), a line enclosed in apostrophes will be printed, and then the value of the variable a will be displayed.
The WriteLn statement can also be used without parameters. In this case, a line consisting of spaces will be printed and the cursor will move to another line. We sometimes need this for a better perception of data input.
Read and ReadLn statements
Recall that the main purpose of computers is to save human labor. Therefore, it is necessary to provide the possibility, once having written a program, to use it repeatedly, each time entering different data. This flexibility in the language is provided by the Read and ReadLn statements. These operators enter information from the keyboard.
General form:
Read(variable, variable...)
ReadLn(variable, variable...)
When executing the Read procedure, the values listed in parentheses are expected. The input data must be separated from each other by spaces. Assignment of values goes in turn.
For example, if the values 53 and X are entered, then when the Read (a, b) statement is executed, the variable a will be assigned the number 53, and the variable X will be assigned the letter X. Moreover, we note that in order to avoid an emergency, you need to correctly determine the data type in the section Var; in our case a:integer and b:char.
There are no particular differences between reading and writing in the use of the Read and ReadLn statements. Often, the ReadLn procedure without parameters is used at the end of the program to delay: until a key is pressed
Note . When you set a screen delay, pay attention to the previous input. If the data was requested by the Read procedure, there will be no delay.
Let's solve a problem in which we consider all possible uses of these procedures.
Task . Find the average of three numbers.
Note . To find the average of several numbers, you need to add these numbers and divide the sum by the number of these numbers.
Type the text of the problem and carefully consider each line. The name of the program Srednee reflects the content of the task. By the way, let's agree that the name of the program and the name of the file that contains this program are the same. Next comes the connection of the Crt module. The Var section describes First, Second, Third as variables of an integer type, and Sum as a real type. The operator section begins with the standard procedure for clearing the screen ClrScr (Clear Screen), which is located in the Crt module. Next, using the Write statement, we display the message ‘Enter the first number’ on the screen, upon receipt of which the user must enter a number.
The computer must now read the entered characters and store them in the First variable, this will happen when the next ReadLn(First) statement is executed. Then, using the Write statement, we request the values of two more numbers and read them into the Second and Third variables. Then we calculate their sum and assign the resulting number to the Sum variable. To find the average, you now need to divide the resulting number by 3 and store the result in some variable.
It is not necessary to declare another variable to store the result. It is possible, as in our program, to divide the value of the variable Sum by 3 and again assign the result to the same variable Sum. Now you can display the result of the calculation on the screen using the Write procedure. And finally, the last procedure ReadLn will delay our output on the screen until the key is pressed.
Press the keys
Average of 5, 7 and 12 is 8.00
Look carefully at this line and compare with the output line of the result in our program. Test the program a few more times for other variable values.
Choose with the teacher tasks to solve from the following list:
- Enter two numbers a and b. Use the assignment operator to exchange their values:
a) using an intermediate variable (x:=a; a:=b; b:=x);
b) without using an intermediate variable (a:=a-b; b:=a+b; a:=b-a). - Write a program that prompts the user for an integer, a real number, an arbitrary character, and a string, and then prints them all on one line.
- Display your last name, first name, and patronymic, and two lines later, your date of birth.
- Write a program to print one of the figures with asterisks:
a) Christmas trees (several Christmas trees);
b) snowflakes (several snowflakes);
c) a house. For example,*
* *
* *
***********
* *
* *
* *
* *
*********** - Compose your business card.
* Ivanov Sergey *
* Proletarian 74 sq. 55*
* Phone 45-72-88 *
******************************* - Compose a dialogue between the user and the computer on an arbitrary topic.
For example, the machine asks two questions “What is your name?” how old are you?"; after entering the name (Anton) and the number (15), it displays “Yes ... In 50 years you will already be 65 years old, and your name will not be Anton, but grandfather Anton” - Ask the user for two numbers and display the result of the sum, difference, product and quotient of these numbers as a full answer.
- Ask the user for two numbers and display the result of the integer division and the remainder of the integer division as a table. For example, when entering the numbers 5 and 3, the following table should appear on the screen:
**************************
*X*Y*div*mod*
**************************
* 5 * 3 * 1 * 2 *
************************** - Write a program that asks for the name of an animal and a number, and then displays a phrase like "The squirrel will eat 10 mushrooms" (when entering the word "squirrel" and the number 10).
- Organize a dialogue between the seller (computer) and the buyer (user) when buying a product according to the following scheme: offering a product at a certain price, requesting the quantity of the purchased product, determining and displaying the amount of money that the buyer must pay for the purchase.
The Pascal programming language uses instructions such as read and readLn. What are they?
What is a read instruction?
This manual is intended to provide input from the PC keyboard different meanings variables when using the Pascal language. The scheme for using the instruction in question looks simple: like read (“variable value”).
In practice, the read statement is used to ensure that certain data is read from a file and then the values extracted from the corresponding data are assigned to the variables that are specified when calling the procedure.
If the user made a mistake when entering data, they do not correspond to any type of variables reflected in the instruction, the program stops executing commands. At the same time, a message appears on the PC screen stating that an error has occurred in the application.
If the programmer uses multiple read instructions, then the data will be entered one way or another on the same line. The transition to the next is possible only if the current line ends. However, you can read information placed on another line using the readLn instruction. Let's consider its features in more detail.
What is the readLn instruction?
The essence of the readLn instruction is to set a condition in the program under which:
- any number entered into the line is assigned to the last variable according to the instruction;
- the remaining area of the line is not processed by the program, while the next instruction will require new input.
So, you can enter the instruction:
readLn(C,D); read(E);
And if after that the row 1 2 3 is entered from the keyboard, then the variable C will acquire the value 1, D - 2. But the program will not assign a specific value to the variable E until the user enters a new number.
As with the read statement, if the user enters a data type incorrectly through the readLn command, the program exits and reports that an error has occurred.
Comparison
The main difference between readLn and read is that the first procedure involves the program jumping to the line of the file following the one in which the instructions are written. The second procedure allows the program to read the data placed on the next line only with the permission of the user - if he presses Enter.
In practice, the use of the readLn instruction is most often used to provide a delay between the result of the application execution and the transition to next instruction. The corresponding delay lasts until the user presses Enter.
Having determined what is the difference between readLn and read in Pascal, let's fix the conclusions in the table.
4. Operators write and writeln. Output procedures
You noticed that the write and writeln statements were used in the program. The English word write is translated - to write, and the word writeln comes as an abbreviation of two English words write - to write and line - a line.
In addition to the write and writeln operators, we are talking about output procedures.
What is procedure ?
The concept of procedure is one of the basic concepts of Pascal. It is similar to a BASIC subroutine.
Procedure is a sequence of Pascal language operators that has a name and can be accessed from anywhere in the main program by specifying its name.
Above, we talked about information output operators, although in Pascal, unlike BASIC, there are no information output operators, and through the write and writeln service words, there is an appeal to standard or built-in information extraction procedure. The standard procedure does not require preliminary description, it is available to any program that contains a call to it. That's why the call to write or writeln resembles the PRINT statement - the output of information in the BASIC language.
Difference between output operator and appeal to withdrawal procedure is that the name of the output procedure, like any other Pascal procedure, is not a reserved word, and, therefore, the user can write his own procedure with the name write or writeln. But this is very rarely used in practice.
Thus, the write and writeln operators are operators for calling built-in output procedures.
Both of these procedures display information on the screen, if this information is contained in the form of variable values, then it is enough to write the names of these variables in brackets in the write or writeln statements, for example: write(a), writeln(f). If there are several such variables, then they are written separated by commas, for example: write(a, b, c, d), writeln(e, f, g, h).
If the information is words, sentences, parts of words or symbols, then it is enclosed between the signs "; " "; - apostrophe, For example:
write("Enter path length"),
writeln("The speed value is"
Simultaneous output and character information and variable values, then in the write or writeln statement they are separated by commas, for example:
write("The temperature value is ", t),
writeln("Speed equals ", v, " with travel time ", t).
Note that there is a space at the end of words before the apostrophe.
What is it made for? Of course, so that the following numerical information is separated from the words by a space.
What does it consist of difference in the work of the write and writeln procedures?
The write procedure requires the following input or output procedures to input or output information on the same line (one line).
If a program specifies a write statement and is followed by another write or writeln statement, then the output from them will be appended to the information line of the first write statement.
For example: write("Today and tomorrow will be ");
write("holidays");
The screen displays:
Today and tomorrow will be days off
Space between the word ";will"; and ";weekend"; provided by a space at the end of the first line. If it does not exist, then the output will occur together :
write("Today and tomorrow will be");
write("holidays");
Today and tomorrow will be days off
Some more examples: t:=20;
write("Movement time is ");
write("seconds");
Movement time is 20 seconds
write("The sum of the numbers is ");
write(", and product ");
The sum of the numbers is 30 and the product is 216
Procedure writelnprovides for the following input or output procedures to enter or output information from the beginning of each new line.
In a programme:
writeln("Tonight, tonight, tonight,");
writeln("When the pilots, let's face it, have nothing to do");
The screen displays:
Tonight, tonight, tonight
When the pilots, frankly, have nothing to do
In a programme:
writeln("The sum and difference of the numbers are:");
On the screen:
The sum and difference of the numbers are:
5. Read and readln statements. Information entry procedures
As well as for information output statements, the read and reeadln statements are operators for accessing built-in information entry procedures.
The operators read (read) and readln, which comes from two English words read (read) and line (line) are used in programs to enter information into the computer's memory and "; readings"; values into a variable.
Consider the work of these operators and procedures for entering information.
Our program has a readln(a) procedure. When executing a program, upon encountering a readln statement, the computer will pause while waiting for information to be entered. After we enter the value of the variable a - 16 from the keyboard, the computer will assign this value to the variable a, i.e. will send it to a memory location named a and continue executing the program. We call this process "; reading"; values into a variable.
So, the procedures read and readln ";read"; variable values and assign them to the variables that are written in them.
There can be several such variables, then they are written in these operators separated by commas, for example:
read(a, b, c, n, g, j, i), readln(e, f, k, p, d) etc.
What is the difference between read and readln procedures?
The read procedure will require input or output of information on one line after itself, and the readln procedure allows you to enter and output information after itself from the beginning of a new line.
For example:
In the program: write("Enter the values a and b "); read(a,b);
write("Entering information in one line");
When this part of the program is executed, everything written in the first write statement will be displayed on the screen, then the cursor will be on the same line, and the computer will wait for the values a and b to be entered. Let's enter their values - 2 and 3, separating them with a space or, in other words, with a space. After that, the information written in the next write statement will be output on the same line.
On the screen:
Enter the values for a and b 2 3 Enter information on one line
In a programme:
writeln("Enter a, b, and c); readln(a, b, c);
writeln("Input and output information from the beginning of the line");
On the screen:
Enter the values a, b and c
Entering and outputting information from the beginning of a line
Chapter 2 Entering and Running Programs
1. In the integrated environment Turbo Pascal 7.0.
After launching Turbo Pascal, the following shell will appear on the screen (see Fig. 3):
Rice. 3
The top line of the opened window contains "; menu"; possible modes of operation of Turbo Pascal, the bottom - a brief note on the purpose of the main function keys. The rest of the screen belongs to the window textual editor, outlined by a double frame and intended for entering and editing the text of the program.
When we enter a program, we work with a text editor built into the Turbo Pascal environment. Therefore, below we will get acquainted with the work of the editor and its main commands.
A sign that the environment is in an editing state is the presence of a cursor in the editor window - a small blinking dash.
To create program text, you need to enter this text using the keyboard, just as it is done when typing text on a typewriter. After filling in the next line, press the key B> Enter> "; Input"; to move the cursor to the next line (the cursor always shows the place on the screen where the next input character of the program will be placed).
The editor window imitates a long and fairly wide sheet of paper, part of which is visible in the screen window. If the cursor has reached the bottom edge, the editor window is scrolled: its content is shifted up one line and a new line appears at the bottom "; sheet";. The longest string in Turbo Pascal is 126 characters.
The window can be shifted relative to the sheet using the following keys:
pgup- page up ( Page UP- page up);
PgDn- page down ( Page Down- page down);
Home- to the beginning of the current line ( HOME- home);
End- to the end of the current line ( END- end);
Ctrl-PgUp- at the beginning of the text;
Ctrl-PgDn- at the end of the text.
Cursor keys “ cursor” can be moved in the text on the screen (note, only in the text!). By "; pure "; on an unwritten screen, the cursor does not move!
If you make a mistake when entering the next character, you can erase it using the key marked with an arrow to the left (key backspace- B>Background>, it is located to the right and at the top of the main alphanumeric keys area above the B>Enter> key - “ Input”). B>Del key ete> (Delete - erase, delete) erases the character to which this moment specifies the cursor, and the Ctrl-Y command indicates the entire line on which the cursor is located. It should be remembered that the Turbo Pascal editor inserts a delimiter symbol invisible on the screen at the end of each line. This character is inserted with the B>Enter> key, and deleted with the B>Backspace> or B>Del key ete> . By inserting/deleting a delimiter, you can "; cut”/";glue"; lines.
To "; cut"; line, move the cursor to the desired location and press the B>Enter> key to "; glue"; adjacent lines, you need to place the cursor at the end of the first line and press the B> Del key ete> or place the cursor at the beginning of the next line and press B>Backspace>.
Insert mode
The normal mode of the editor is the insert mode, in which each newly entered character is like "; pushes apart"; text on the screen, shifting the rest of the line to the right. Note that "; cutting"; and subsequent insertion of missing characters is possible only in this mode.
Examples "; cutting";, ";gluing"; strings and inserting characters into text.
Suppose, for some reason, the following entry is received on the screen:
program Serg; var
a, b, c: integer;
If we talk about the aesthetic side of writing a program, then it is desirable that the section of descriptions that begins with the word var, started with a red line. To make changes to the text, place the cursor on the letter v and press the B>Enter> key, while the part of the text after the cursor and below it will move to the next line, we get:
program Serg;
a, b, c: integer;
For greater beauty and clarity, without moving the cursor, but leaving it on the letter v, press the B>Space> key several times. The whole line will move to the right and the entry will become:
program Serg;
a, b, c: integer;
Let's say another situation, when part of the text "; broke"; and we need it"; glue";, for example, it turned out like this:
write("Enter the number of years that
swarm would be Serezha ";);
Set the cursor to the beginning of the second line before the letter ";p"; and press the key B>Backhole>, we get:
write("Enter the number of years that would be Serezha");
You can do otherwise, place the cursor at the end of the first line after the letter ";o"; and pressing several times the B>Delete> ";pull up"; bottom line up.
Using the keys B>backspace> and B>Del ete> Can "; unite"; ";torn” string. For example, in this situation:
write("Enter the number of years that would be Serezha");
Set the cursor before the letter ";d"; and press the key B>backspace> several times until the word ";Enter"; will accept the desired construction, or by placing the cursor after the letter "e"; press the B>Delete> key several times.
Inserting missing characters is made even easier.
For example, you missed a few letters:
wrte("Enter the number of years that would be ");
The letter ";i"; is missing in the first word, in the word ";Enter";; missing two letters ";di";, in the word ";Seryozha"; letters ";er";.
Set the cursor to the letter ";t"; in the first word and type ";i"; from the keyboard, it will immediately be inserted into the right place. Next, set the cursor to the letter "t"; in the word ";Vvete"; and type from the keyboard ";di";, the word "; will move apart"; and the letters "; di"; will fall into place. Place the cursor on "; e"; in the word "; Sezha"; and type "; er";,
Blend Mode
The editor can also work in the mode of superimposing new characters on existing old text: in this mode, the new character replaces the character pointed to by the cursor, and the rest of the line to the right of the cursor is not shifted to the right. To switch to blend mode, press B>Ins ert> (Insert- Insert), if you press this key again, the insert mode will be restored. An indication of which mode the editor is in is the shape of the cursor: in insert mode, the cursor looks like a blinking underscore, and in overlay mode, it's a large, blinking rectangle that obscures the entire character.
Auto indent mode
Another feature of the editor is that it usually works in auto-indent mode. In this mode, each new line starts at the same screen position as the previous one.
The auto-indent mode maintains a good style for the design of program texts: indents from the left edge highlight various operators and make the program more visual.
You can disable the auto-indent mode with the command Ctrl-O I(when the key is pressed ctrl key is pressed first O, then key O released and the key is pressed I), repeated command Ctrl-O I restore auto-indent mode.
The most commonly used commands are listed below. text editor Turbo Pascal other than those listed above.
Editing Commands
backspace- B>Backhole> - delete the character to the left of the cursor;
Del- erase the character pointed to by the cursor;
ctrl-y- erase the line on which the cursor is located;
Enter- B>Input> - insert a new line, "; cut"; old;
Ctrl-Q L- restore current line(valid if
the cursor did not leave the modified line).
Working with the block
Ctrl-K B- mark the beginning of the block;
Ctrl-KY- delete the block;
Ctrl-K V- move the block;
Ctrl-K P- print the block;
Ctrl-K H- hide/show the block (uncheck);
Ctrl-K K- mark the end of the block;
Ctrl-K C- copy block;
Ctrl-KW- write block to disk file;
Program execution
After the program is typed, you can try to execute it.
To do this, press the keys B>Ctrl>+ (while holding the key B>Ctrl>, press the key B>F9>). The same operation can be performed by going to the main menu, pressing the B>F10> key, and then moving the pointer to select the option Run and press B>Enter>.
The second level menu associated with this option will open on the screen. The new menu is like "; falls out"; from the top line, so such a menu is often called a drop-down (Pull-down). The screen will become like this (see Fig. 4):
Rice. 4
Now you need to find the option in the new menu RUN(start) and press B>Enter>.
If there was no error when entering text, then, after a few seconds, the image on the screen will change. Turbo Pascal puts the screen at the disposal of the running user program. This screen is called program window.
In response to a request:
Enter the number of years that would be Serezha, you must enter 16 and press the B>Enter> key.
After the run is completed (the program's work is often called its run), the editor window with the program text will reappear on the screen. If you did not have time to see the image of the program window, then press Alt-F5. In this case, the editor window disappear and you can see the results of the program. To return the screen to the playback mode of the editor window, you must press any key.
You can make the screen more convenient to see the results of the program. To do this, you can open a second window at the bottom of the screen.
To do this, press the F10 key to enter the selection mode from the main menu, hover over the option Debug(debug) and press the B>Enter> key - the second level menu associated with this option will open on the screen. The screen will look like this (see Figure 5):
Rice. 5
Find the option OUTPUT (program output) in the new menu, move the pointer to it and press the B>Enter> key.
A second window will appear at the bottom of the screen, but it will no longer disappear.
Now let's make sure that two windows are shown on the screen at the same time: press the F10 key again, select WINDOW, press B>Enter>, move the pointer to the option TILE(tiling) and press B>Enter>.
If everything is done correctly, the screen will look like (see Fig. 6):
Rice. 6
A double frame that outlines the program window indicates that this particular window is currently active.
Let's make the editor window active: press the B>Alt> key and, without releasing it, the key with the number 1 (the editor window has the number 1, the program window has the number 2, these numbers are written in the upper right corners of the frames). Now everything is ready for further work with the program.
First mistakes and their correction
1. No semicolon, for example, after the readln(a) statement. After starting the program, by pressing the keys B>Ctrl>+B>F9>, in top line The following message will appear on the screen, written in red:
Error 85: ";;"; expected.
(Error 85: ";;"; missing.)
The editor will set the cursor to the next character after the missing character, in our example the b variable. After pressing any key, the error message disappears and the editor goes into insert mode. It is necessary to move the cursor to the desired place, put a semicolon - “;” and continue working.
2. A variable is not written in the description of variables, but it is present in the program, for example, a variable c. After starting the program, the following message will be displayed:
Error 3: Unknown identifier.
(Error 3: Unknown identifier.)
The cursor will be placed on this variable, in our example on the variable c. It is necessary to correct the error, i.e. write variable c to the variable declarations section and continue working.
3. Not put a dot after the operator end at the end of the program. The compiler message will be:
Error 10: Unexpected end of file.
(Error 10: Wrong end of file.),
the cursor will be positioned on the letter "; e"; in a word "; end";. You need to put a period and run the program again.
Writing a file to disk
So, the program has been edited and executed (scrolled), now it needs to be written to disk. To do this, you can use the main menu, in which select the option "; file"; (see Fig. 7). The sequence of actions is as follows: 1) press the F10 key and go to the main menu; 2) move the pointer to the option "; file"; and press B>Enter>, the second menu of the option "; file";:
Rice. 7
You can select from this menu the option "; Save";. It writes the contents of the active editor window to a disk file.
If you press B>Enter>, the environment prompts for a file name if one was not set and the window was associated with the name NONAME00.PAS. You can change the name, or leave it the same.
This option is called directly from the editor by pressing B>F2>.
You can choose an option SAVEAS. It writes the contents of the active editor window to a disk file under a different name.
The dialog box for this option looks like (see Figure 8):
Rice. 8
In the input field, you must write the name of the file into which the contents of the active editor window will be rewritten. You can select an already existing file from the selection field or from the protocol with options. In this case, depending on the environment setting, the old contents of the file will be destroyed or saved as a backup copy with a .BAK extension.
Line-by-line writing of program text
There are no rules in Pascal for splitting program text into lines.
However, to write the program, you can give some
It is very important that the text of the program be arranged visually, not only for the sake of beauty, but (and this is the main thing!) for the sake of avoiding errors. (Finding errors in visual text is much easier.)
1. Each statement should be written on a new line, with the exception of short and meaningfully related statements.
For example,
write ... readln ... are written on one line, short assignment statements can be written on one line:
a:= 23; b:= 105; c:= -11.2.
2. Operators of the same level located in different lines must be aligned vertically, i.e. equally spaced from the left edge.
For example, let's write a sequence of operators to determine the sum of the digits of a three-digit number:
s:= a div 100;
d:= a div 10 mod 10;
e:= a mod 10;
Here, all operators are equivalent, they follow one after the other in sequence, so they all start from the same vertical position.
3. Operators included in another operator should be shifted to the right by several positions (preferably the same).
if ... then
4. It is recommended to vertically align pairs of base words: begin And end, with which we have already met, as well as words that we will meet later: repeat And until, record And end, case And end.
Here are some of the most popular operator accommodation options if:
A) if ... then ...
else ...
b) if ... then ...
else ...
V) if...
then ...
else ...
G) if ...
then ...
else ...
e) if ... then ... else ...
6. Comments are written either next to the construction (identifier, operator, part of it), which they explain, or in a separate line.
Documentprogramming programming programming, general ... Coffin silence. › Several times paired programming gone...
Alistair cowburn pair programming advantages and disadvantages
DocumentRelated research programming and organizational efficiency. Amazing... language programming, certain design methods and programming, general ... Coffin silence. › Several times paired programming gone...
An Introduction to Neuro-Linguistic Programming The Latest Psychology of Personal Excellence
DocumentWill it be called?" The result was Neurolinguistic programming- a cumbersome phrase that hides ... taciturn, vocal, sound, voice, says, silence, dissonance, consonant, harmonious, shrill, quiet ...
NEUROLINGUISTIC PROGRAMMING (guide for beginners)
DocumentPSYCHOTHERAPEUTIC CENTER "LAD" V.I.ELMANOVICH NEUROLINGUISTIC PROGRAMMING(guide for beginners) PART1. ... MODALITIES (A). 1. If volume = 0, then “listens silence", if the volume is maximum, then “on ...